What's new
Handwriting to text in seconds: Meet Evernote's AI Transcribe
Capture, upload, and turn handwriting into editable, searchable text—instantly.
Read more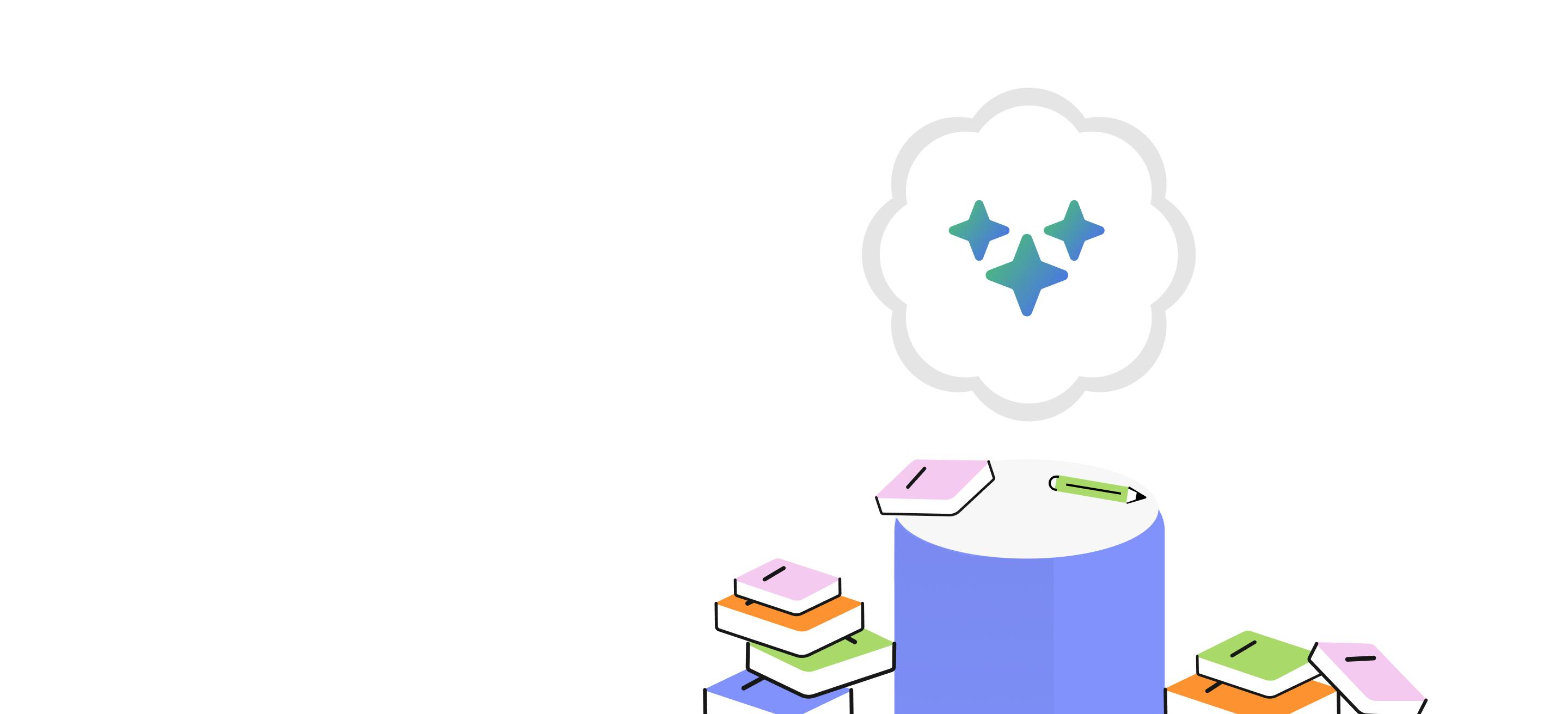
Curious about what's happening at Evernote? Check out the articles below to see all the exciting things we're working on.
Capture, upload, and turn handwriting into editable, searchable text—instantly.
Read moreSkip the replay—convert any video, audio, or link to text in seconds with AI.
Turn your recordings, screenshots, and scribbles into clean, editable text in just a few clicks.
From voice memos to lecture videos to notebook pages—turn it all into text, in seconds.